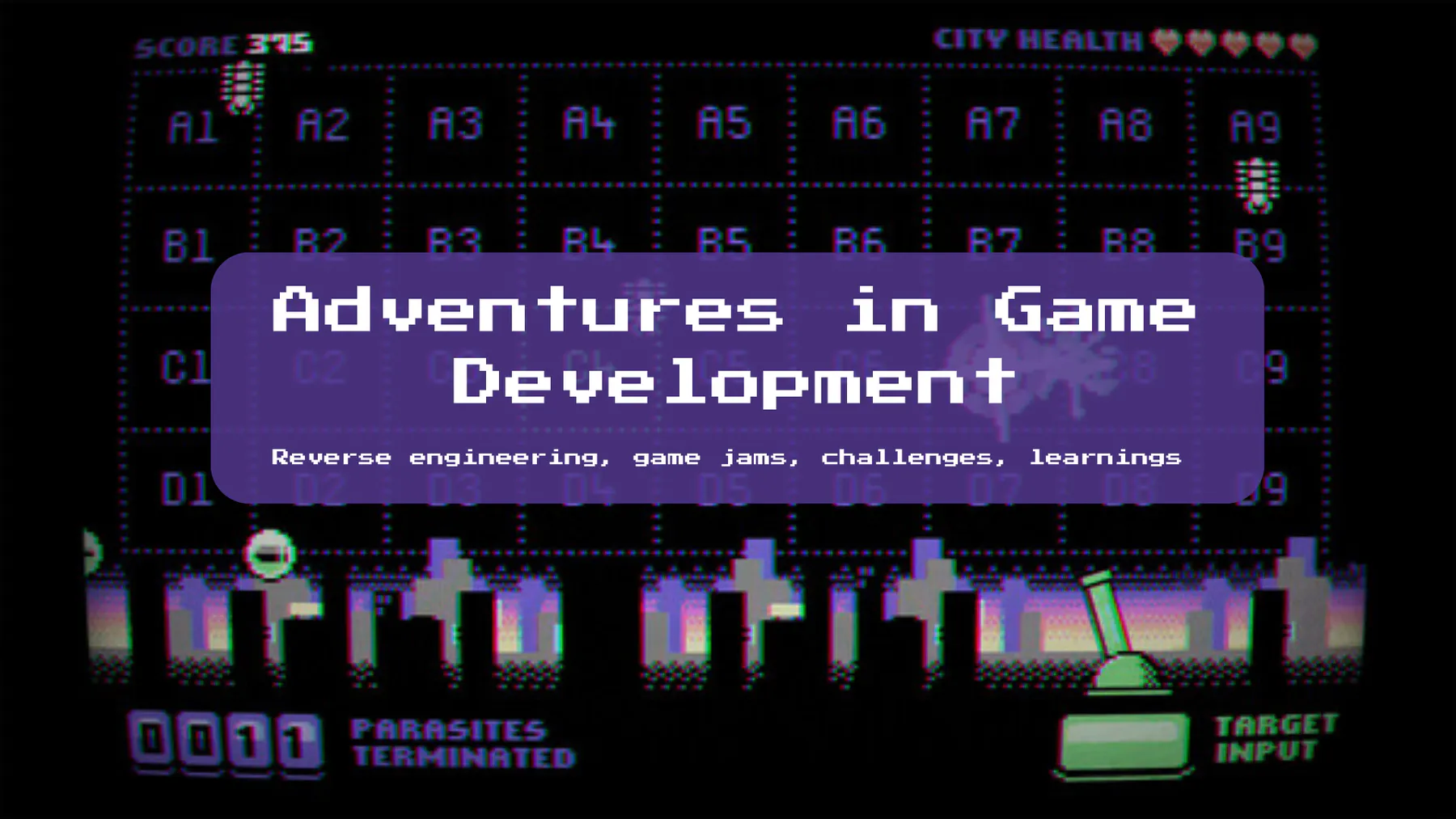
Adventures in Game Development
Reverse engineering, game jams, challenges, learnings
Reverse engineering, game jams, challenges, learnings
Reverse engineering, game jams, challenges, learnings
Blazingly fast and hosted for free on the edge
UNRECORD is causing a stir on social media
A short and simple guide
A Modern Approach to Legacy Web Applications
Now with more ES6
A change of scenery and means
Trying out three XBMC distributions